Are you wrestling with the challenge of implementing input masks in your Django forms, only to find yourself facing a string of unsuccessful attempts? The ability to effectively mask input fields is crucial for data validation and user experience, and the correct approach can transform a frustrating experience into a seamless one.
The initial hurdle often lies in the integration of the chosen masking library or widget. One might begin by importing the necessary components, such as `inputmask.widgets` and then `inputmask`, but the desired result remains elusive. Perhaps, due to the complexities involved, the implementation doesnt achieve the desired outcome. The underlying issue might stem from a misunderstanding of how the masking library interacts with the form fields or from overlooking specific configuration settings. This is common for both novice and experienced developers.
Let's examine some potential areas for troubleshooting and ensure a smooth process of masking data entry within your Django project:
Problem Area | Possible Solutions |
---|---|
Incorrect Library Import | Double-check the import statements. Make sure you're importing the correct widgets and classes. Verify that the masking library is installed correctly in your project environment (e.g., using pip). |
Widget Application | Ensure that the masking widget is correctly assigned to the form field. This is done by specifying the `widget` attribute in your form field definition. For instance: `Phone = brphonenumberfield(widget=brphonenumberinput)` |
Mask Configuration | Review the masking options or parameters. Input masks often have specific settings to define the mask format, characters allowed, and other behaviors. Refer to the library's documentation for proper configuration options. |
JavaScript Integration | Some masking libraries rely on JavaScript to function correctly on the client side. Ensure that any necessary JavaScript files are included in your template. Also, verify that the JavaScript is being loaded and initialized correctly. |
Form Field Type | Confirm that you're using a form field type that's compatible with the mask you want to apply. For example, a phone number mask is designed for a `CharField` or a dedicated phone number field like `brphonenumberfield`. |
Conflicting Libraries | Check for any conflicts between different JavaScript libraries or masking plugins. Ensure that the required resources are not overwritten or unintentionally interfered with by other code. |
Browser Compatibility | Confirm that the chosen input masking solution is compatible with the target browsers your users will use. Sometimes, browser-specific adjustments or workarounds may be necessary. |
Consider a simple Django app to mask sensitive data in forms. In addition, data masking is a powerful tool. It protects sensitive data by name of form fields and is highly configurable. It could also be an application for masking. Data masking is essential for security and privacy.
Let's consider a concrete example within a Django context. Suppose you are building a form where users need to enter a phone number. You want to ensure the number is entered in a specific format (e.g., (XXX) XXX-XXXX). The recommended approach involves the following steps:
- Installation: First, you'll need to install an input masking library. One such library offers Django integration. Install it via pip: `pip install django-input-mask`.
- Form Definition: In your `forms.py` file, define your form and include the masked field:
from django import formsfrom input_mask.widgets import InputMaskclass YourForm(forms.Form):phone_number = forms.CharField(widget=InputMask(mask="(999) 999-9999"))
- Template Integration: In your template, ensure that the form is rendered correctly, and the necessary JavaScript and CSS are loaded. This might involve including the library's static files.
Remember that the specific syntax and implementation details may vary depending on the chosen input masking library. Always consult the library's documentation for the most accurate and up-to-date instructions. The use of a dedicated phone number field (`brphonenumberfield`) with an associated widget (`brphonenumberinput`) can streamline the process further, providing a more robust and feature-rich solution.
Delving into the world of Django and JavaScript, it's worth noting a pro-tip by CaioAriede. This is a reminder of the dynamic interplay between backend and frontend in modern web development. The correct application of input masks enhances user experience. It also helps enforce data integrity, making it a crucial aspect of form design.
The focus is on the technical aspects of form design and data validation, the topic of "Django unchained" can come into play.
Consider the "baghead" scene from Quentin Tarantino's Django Unchained. It's a conversation among KKK members where the masks fail to conceal identities effectively. This scene, almost cut by Tarantino, serves as a stark reminder that the presentation of the mask can sometimes be as important as its function.
The concept of masking is not just restricted to data entry; it also applies to security, privacy, and visual representation. For example, the data is masked by a simple Django app to mask sensitive data in forms. Data masking is a great tool to mask sensitive data by name of form fields, and it is highly configurable.
The film itself, with Jamie Foxx, Christoph Waltz, Leonardo DiCaprio, and Kerry Washington, explores themes of identity, deception, and the consequences of masks, both literal and figurative. The way the camera lingers on certain characters during key scenes suggests there was initially a deeper layer of narrative intended. This is especially true for Zoe Bells masked character, which was easily one of the most confounding parts of Django Unchained. The question is always, what was up with her?
From the perspective of the film, consider the following table:
Movie Title | Django Unchained |
---|---|
Director | Quentin Tarantino |
Main Actors | Jamie Foxx, Christoph Waltz, Leonardo DiCaprio, Kerry Washington |
Themes | Slavery, Revenge, Freedom, Identity |
Memorable Scenes | "Baghead" scene, Candyland scenes, Django's pursuit of Broomhilda. |
Production | Quentin Tarantino / produced by: Stacey Sher / Reginald Hudlin/ Pilar Savone / written by: Jamie Foxx / Christoph Waltz / Leonardo |
Release Year | 2012 |
Key Locations | Louisiana, Candyland (fictional plantation) |
Iconic Dialogue | "I like the way you die, boy." |
Music | Ennio Morricone, Various |
Reference: IMDb
The "baghead" scene, despite its initial potential for removal, is now iconic. It is also a case in point. It reveals the absurdities of racism through the lens of the characters' inability to properly execute their disguise. In the film, some characters' lines expose their ignorance and prejudice, and the audience understands the situation.
The masks, in this context, are both literal and metaphorical. They represent the facade of authority and superiority the KKK members attempt to maintain, which is constantly undermined by their incompetence and the absurdity of their views.
The importance of a well-crafted mask in various contexts and the way the film subverts expectations.
The film's use of masks to explore themes of identity and disguise. It also explores the consequences of prejudice and the struggle for freedom.
The film's exploration of disguise, deception, and the consequences of masks extend beyond the literal. Djangos journey, for instance, involves a series of disguises and pretenses. He must carefully navigate the world of the Candie plantation.
The film's plot takes Django, accompanied by Dr. Schultz, on a journey across America. Their primary mission is to hunt down the brittle brothers and the notorious Calvin Candie, who is the owner of Broomhilda (played by Kerry Washington). The stakes are high, and the environment is fraught with danger. The characters must rely on wit, strategy, and cunning to survive.
The ability to effectively mask input fields is crucial for data validation and user experience. The right approach can transform a frustrating experience into a seamless one.
For developers, whether seasoned or just starting out, grappling with input masks in Django forms can be challenging. The key lies in understanding the intricacies of the chosen libraries or widgets, carefully configuring the mask parameters. With this knowledge, the goal of implementing an effective solution is easily achieved.
Quentin Tarantino reveals that he almost cut the iconic baghead conversation among KKK members in Django Unchained.
In the world of Django, form validation and user experience are intertwined. The implementation of input masks is critical for both.
"Well, make your own goddamn mask!" - This line and others like it, highlight the core of a user's experience with both the movie and the form.
This article serves as a guide to implementing masking in Django forms. It explores the parallels between the technical aspects of the film and the software development process.
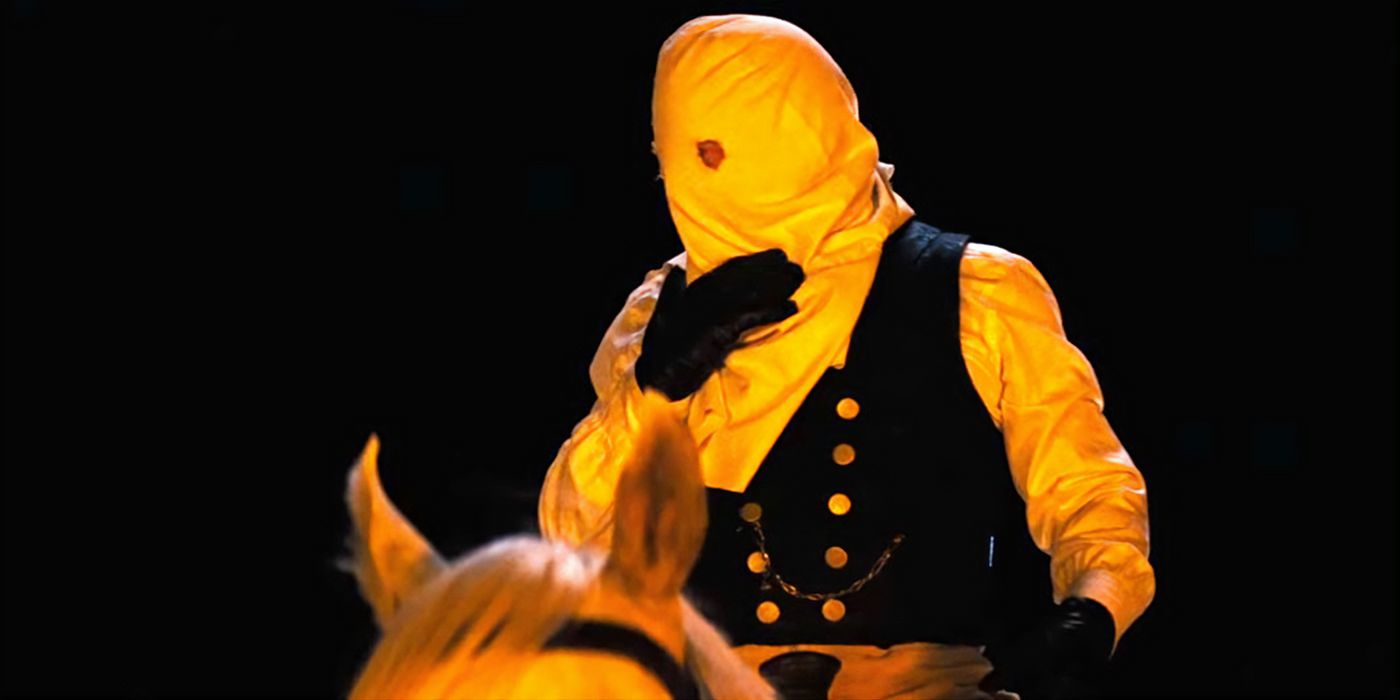

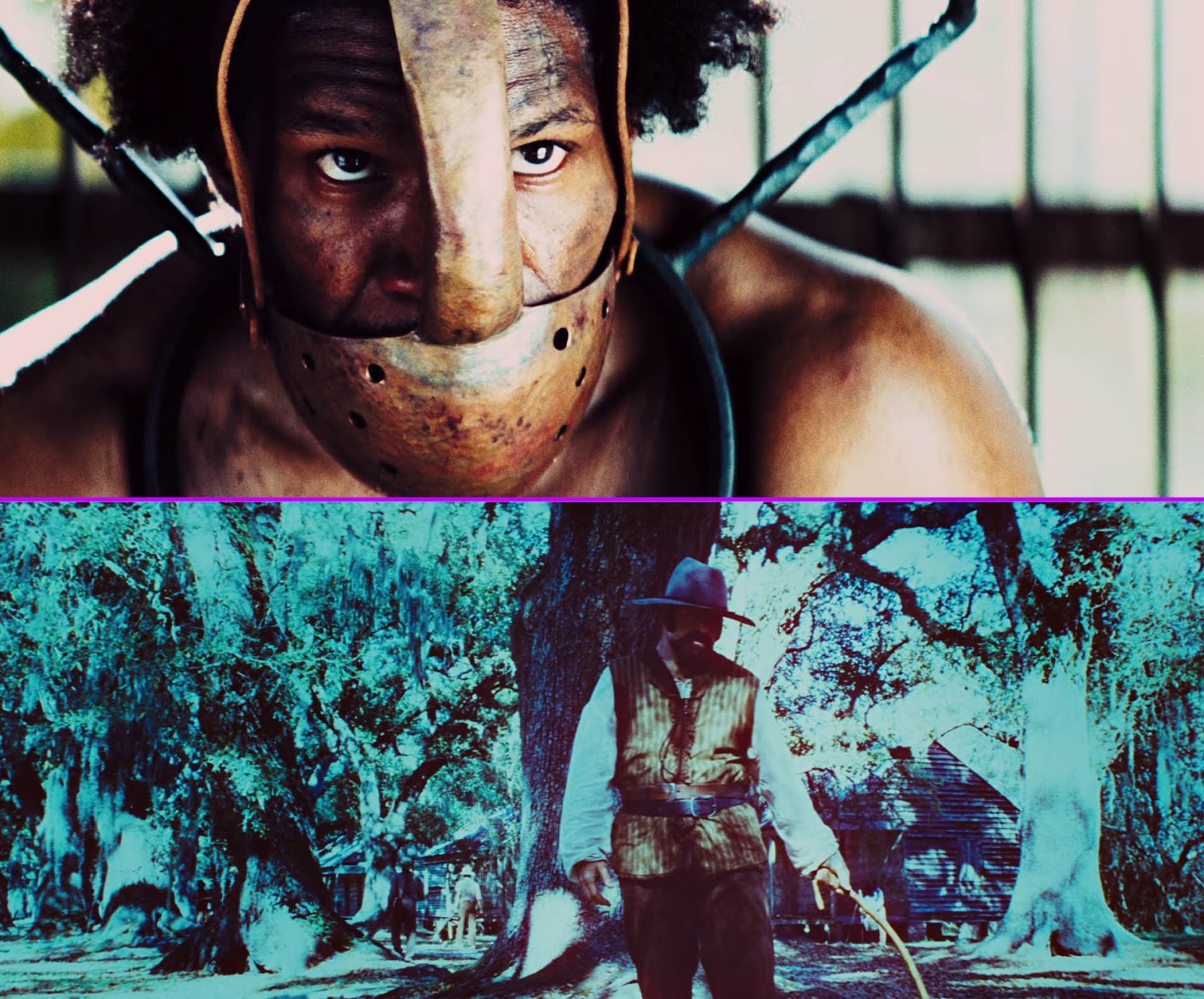